White-Labeling in SurveyJS Survey Software: Customize the appearance of a fully integrated form builder to adhere to corporate identity standards
In today's competitive business environment, the ability to deliver customized solutions to clients is fundamental. White-labeling involves taking a product or service developed by one company and rebranding it to make it appear as if it were created by another company. This practice allows businesses to expand their offerings quickly and cost-effectively. For software like SurveyJS Form Builder, white-labeling means customizing the interface, branding, and sometimes even the functionality to align with a company's brand identity.
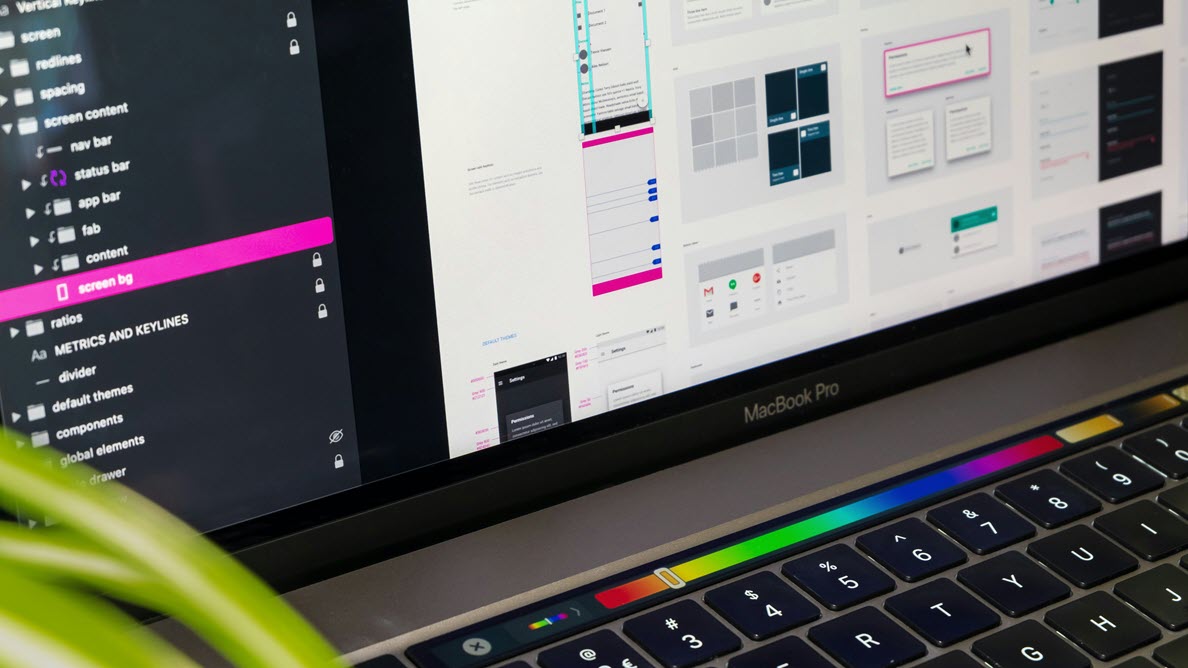
How to White-Label SurveyJS Form Builder
White-labeling for SurveyJS Form Builder involves its integration into your surveying platform and UI customization.
Integrate SurveyJS Form Builder
To integrate SurveyJS Form Builder with your environment, follow the steps below:
Add the Form Builder component to your application
SurveyJS Form Builder supports React, Angular, Vanilla JavaScript, and Vue.js. Follow the instructions given in a Get Started for your JavaScript framework.Host Form Builder on a company-owned domain
Hosting Form Builder on your own domain reinforces your brand's identity by associating the tool with the website it's presented on.Configure user authorization and authentication
Determine the identity of the user who sends a request and the resources they can access. Prevent users from accessing API operations outside their predefined roles.Set up a data storage
SurveyJS Form Builder produces survey configurations (schemas) in JSON format. Use the Form Builder API to load and save the survey schemas from and to a proprietary data storage.
Customize UI Elements
Form Builder UI elements should align with your business requirements. Here are some of the customizations that can help you achieve the desired appearance and behavior:
Add or remove form builder tabs
Hide properties from the Property Grid
Limit available question types
Add custom survey element settings
Customize survey elements on creation
Localize UI Texts
In SurveyJS Form Builder, UI texts are defined within the current locale, which is specified by the locale
property. The default locale is English. To customize UI texts, update the localization dictionary that corresponds to the current locale.
Change the Color Scheme
SurveyJS Form Builder uses SCSS variables to colorize its UI elements. A complete list of variables is available in the variables.scss file. To change a default color scheme, create a style sheet that overrides corresponding SCSS variables and apply it Form Builder. Additionally, you can replace default icons with custom icons.
Use Case: Implementing a Quiz Designer with a Color Scheme Toggle
In this use case, we'll customize SurveyJS Form Builder to function as a quiz editor. Key steps include:
- Limit available question types to Single-Line Input, Radio Button Group, Rating Scale, and Yes/No questions.
- Hide all tabs except Designer and Preview.
- Restrict available settings as necessary.
- Replace occurrences of "survey" with "quiz" wherever applicable.
- Implement a color scheme toggle to enable dark mode.
Limit Available Question Types
To restrict available question types, define the questionTypes
array and list the desired question types. The code snippet below enables the following question types:
- Single-Line Input (
"text"
) - Radio Button Group (
"radiogroup"
) - Rating Scale (
"rating"
) - Yes/No Question (
"boolean"
)
import { SurveyCreatorModel } from "survey-creator-core";
const options = {
questionTypes: ['text', 'radiogroup', 'rating', 'boolean']
};
const quizBuilder = new SurveyCreatorModel(options);
Limit Available Tabs
By default, SurveyJS Form Builder enables three tabs: Designer, Preview, and JSON Editor. You can manage the availability of each tab using the ICreatorOptions
configuration object. To hide the JSON Editor tab, disable the showJSONEditorTab
property:
import { SurveyCreatorModel } from "survey-creator-core";
const options = {
// ...
showJSONEditorTab: false
};
const quizBuilder = new SurveyCreatorModel(options);
Restrict Available Settings
Use the onPropertyShowing
event to specify whether a property is available in the UI. The following array lists properties that should be hidden:
import { SurveyCreatorModel } from "survey-creator-core";
const propertyStopList = [ 'visibleIf', 'enableIf', 'requiredIf', 'bindings', 'defaultValueExpression', 'columnsVisibleIf', 'rowsVisibleIf', 'hideIfChoicesEmpty', 'choicesVisibleIf', 'choicesEnableIf', 'minValueExpression', 'maxValueExpression','calculatedValues', 'triggers', 'resetValueIf', 'setValueIf', 'setValueExpression',
];
const quizBuilder = new SurveyCreatorModel(options);
quizBuilder.onPropertyShowing.add((_, options) => {
options.show = propertyStopList.indexOf(options.property.name) === -1;
});
Update UI Texts to Replace "survey" with "quiz"
Form Builder's UI strings are defined within the current locale. To customize texts used in the designer, access the target translation dictionary and override localization strings. Alternatively, you can create a custom locale to apply multiple translations in a batch. For detailed instructions, refer to the following help topic: Localization & Globalization.
To customize translations, retrieve the translation dictionary object using the getLocaleStrings(localeCode)
method and customize individual properties. The following code snippet replaces instances of "survey" with "quiz" in various translation strings:
import { getLocaleStrings } from "survey-creator-core";
const englishLocale = getLocaleStrings("en");
englishLocale.ed.survey = 'Quiz';
englishLocale.ed.settings = 'Quiz Settings';
englishLocale.ed.surveySettingsTooltip = 'Open Quiz Settings';
englishLocale.ed.surveyTypeName = 'Quiz';
englishLocale.ed.testSurveyAgain = 'Run Quiz Again';
englishLocale.ed.saveSurvey = 'Save Quiz';
englishLocale.ed.surveyResults = 'Quiz Results';
englishLocale.ed.surveyPlaceHolder =
'The quiz is empty. Drag an element from the toolbox or click the button below.';
englishLocale.pe.surveyTitlePlaceholder = 'Quiz Title';
englishLocale.pe.timeLimit = 'Time limit to finish the quiz (in seconds)';
To view a full list of default English strings, refer to the english.ts file. Other languages are available within the localization directory.
Implement a Color Scheme Toggle
To add a checkbox that enables the dark theme for the quiz builder, follow these steps:
- Add a checkbox element to your component's markup.
- Add an event handler for the checkbox to toggle the dark theme.
- Apply the dark theme CSS class to the
<div>
element with the quiz builder when the checkbox is checked.
The following code snippet for React illustrates all three steps:
import { useState } from 'react';
// ...
function QuizBuilderComponent() {
const [isDarkTheme, setIsDarkTheme] = useState(false);
// ...
const handleThemeChange = (event) => {
const isChecked = event.target.checked;
setIsDarkTheme(isChecked);
const quizBuilder = document.getElementById('quiz-editor');
if (quizBuilder) {
if (isChecked) {
quizBuilder.classList.add('darkTheme');
} else {
quizBuilder.classList.remove('darkTheme');
}
}
};
// ...
return (
<div id="quiz-editor">
<label className="custom-checkbox-label">
<input
type="checkbox"
onChange={handleThemeChange}
className="custom-checkbox"
/>
Enable Dark Theme
</label>
<SurveyCreatorComponent creator={quizBuilder} />
</div>
);
}
The dark color scheme is defined by the following CSS class:
.darkTheme {
--primary: #1ab7fa;
--primary-light: rgba(26, 183, 250, 0.1);
--foreground: #ededed;
--primary-foreground: #ffffff;
--secondary: #1ab7fa;
--background: #555555;
--background-dim: #4d4d4d;
--background-dim-light: #4d4d4d;
}
View Full Code
import { useState } from 'react';
import { SurveyCreator, SurveyCreatorComponent } from 'survey-creator-react';
import 'survey-core/survey.i18n.min.js';
import 'survey-creator-core/survey-creator-core.i18n.min.js';
import 'survey-core/survey-core.min.css';
import 'survey-creator-core/survey-creator-core.min.css';
import './index.css';
import { surveyJson } from './surveyJson';
import { getLocaleStrings } from 'survey-creator-core';
import { surveyLocalization } from 'survey-core';
// Replace 'survey' with 'quiz' in UI texts
const englishLocale = getLocaleStrings('en');
englishLocale.ed.survey = 'Quiz';
englishLocale.ed.settingsTooltip = 'Open Quiz Settings';
englishLocale.ed.surveySettingsTooltip = 'Open Quiz Settings';
englishLocale.ed.surveyTypeName = 'Quiz';
englishLocale.ed.testSurveyAgain = 'Run Quiz Again';
englishLocale.ed.saveSurvey = 'Save Quiz';
englishLocale.ed.surveyResults = 'Quiz Results';
englishLocale.ed.surveyPlaceHolder =
'The Quiz is empty. Drag an element from the toolbox or click the button below.';
englishLocale.pe.surveyTitlePlaceholder = 'Quiz Title';
englishLocale.pe.timeLimit = 'Time limit to finish the quiz (in seconds)';
englishLocale.pe.locale = 'Quiz language';
// Restrict available locales
surveyLocalization.supportedLocales = ['en', 'de', 'es', 'fr'];
function QuizBuilderComponent() {
const [isDarkTheme, setIsDarkTheme] = useState(false);
const options = {
questionTypes: ['text', 'radiogroup', 'rating', 'boolean'],
showJSONEditorTab: false
};
const quizBuilder = new SurveyCreator(options);
const propertyStopList = [
'visibleIf',
'enableIf',
'requiredIf',
'bindings',
'defaultValueExpression',
'columnsVisibleIf',
'rowsVisibleIf',
'hideIfChoicesEmpty',
'choicesVisibleIf',
'choicesEnableIf',
'minValueExpression',
'maxValueExpression',
'calculatedValues',
'triggers',
'resetValueIf',
'setValueIf',
'setValueExpression',
];
// Restrict available survey element settings
quizBuilder.onPropertyShowing.add((_, options) => {
options.show = propertyStopList.indexOf(options.property.name) === -1;
});
quizBuilder.JSON = surveyJson;
const handleThemeChange = (event) => {
const isChecked = event.target.checked;
setIsDarkTheme(isChecked);
const quizBuilder = document.getElementById('quiz-editor');
if (quizBuilder) {
if (isChecked) {
quizBuilder.classList.add('darkTheme');
} else {
quizBuilder.classList.remove('darkTheme');
}
}
};
return (
<div id="quiz-editor">
<label className="custom-checkbox-label">
<input
type="checkbox"
onChange={handleThemeChange}
className="custom-checkbox"
/>
Enable Dark Theme
</label>
<SurveyCreatorComponent creator={quizBuilder} />
</div>
);
}
export default QuizBuilderComponent;