Build Dynamic Forms in Blazor with SurveyJS
Blazor is a versatile framework for building dynamic web applications with C#. By combining it with SurveyJS UI components, you can create a fully integrated JSON-based form management system to build and render forms, export them as PDF files, and visualize survey data in custom dashboards. SurveyJS form-building library called Survey Creator seamlessly integrates with Blazor, allowing users to build high-performance, interactive forms using a drag-and-drop UI. This article will guide you through integrating SurveyJS libraries into your Blazor application.
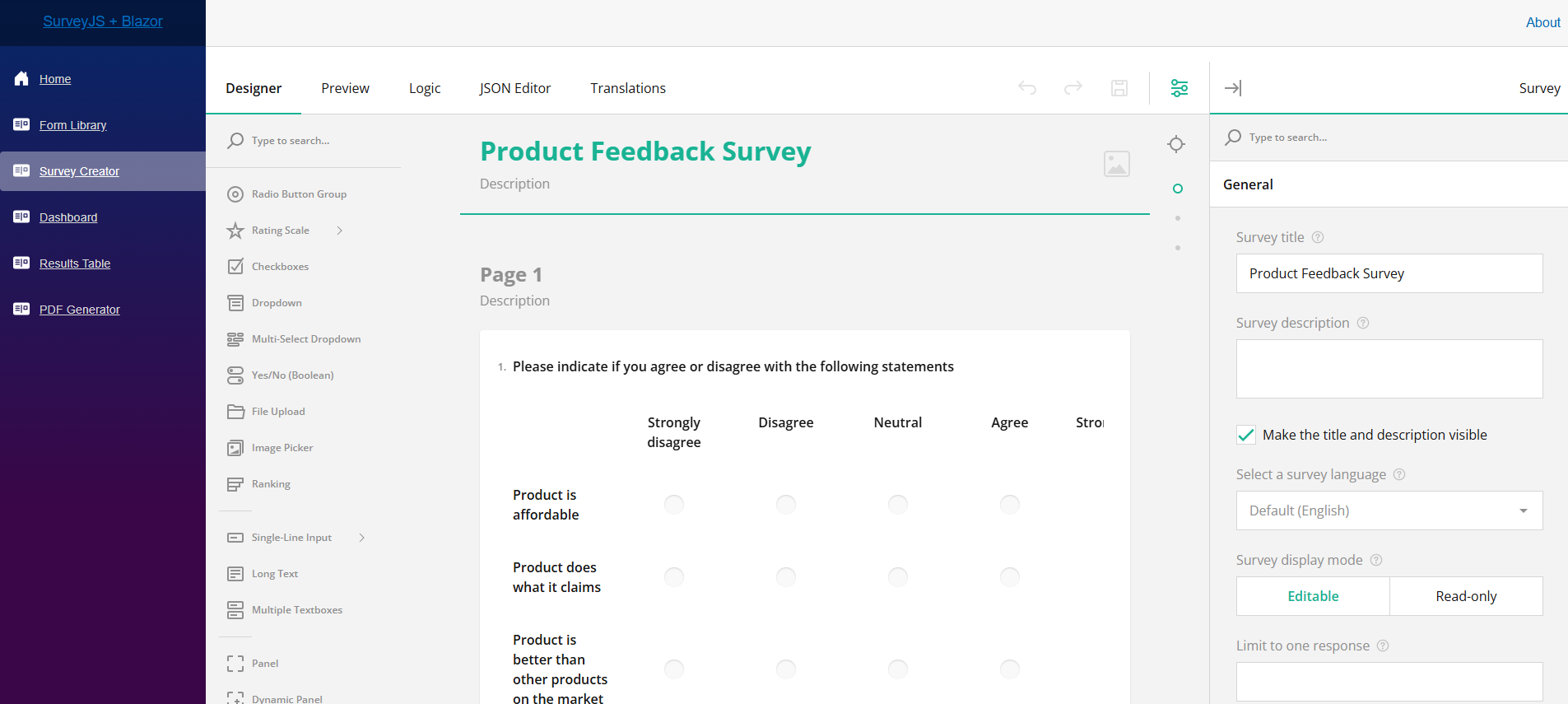
Why Choose SurveyJS for Blazor Dynamic Forms?
SurveyJS is an ideal choice for building forms in Blazor applications. Here's why:
Drag-and-drop form designer
Create forms easily in a visual interface.Conditional logic
Show or hide questions based on user input, allowing for dynamic form adjustments.Extensive input types
Use 20+ accessible fields to create user-friendly forms.Appearance customization
Modify the visual design (fonts, colors, element sizes, etc.) of your forms to align with your application's branding.Customizable components
Implement custom question types to meet specific business requirements.
How to Get Started with SurveyJS in Blazor
Refer to the following GitHub repository to find a demo illustrating how to integrate SurveyJS libraries into a Blazor app.
SurveyJS + Blazor Quickstart Template
Follow these steps to run the demo on your machine:
Clone the repository.
git clone https://github.com/surveyjs/surveyjs-blazor
Navigate to the application's folder.
cd surveyjs-blazor
Install dependencies.
npm i
Run the application.
dotnet run
Open
http://localhost:5208/
in your web browser.
Use the left-side menu to navigate between the different modules of the demo form management system:
This demo showcases SurveyJS components for React, but SurveyJS also provides components for Angular and Vue.js. With other JavaScript frameworks, such as Svelte, you can use platform-agnostic SurveyJS packages designed for vanilla JavaScript, HTML, and CSS.
Integrate Form Library
SurveyJS Form Library is a free MIT-licensed UI component that enables you to render dynamic JSON-driven surveys within your Blazor application.
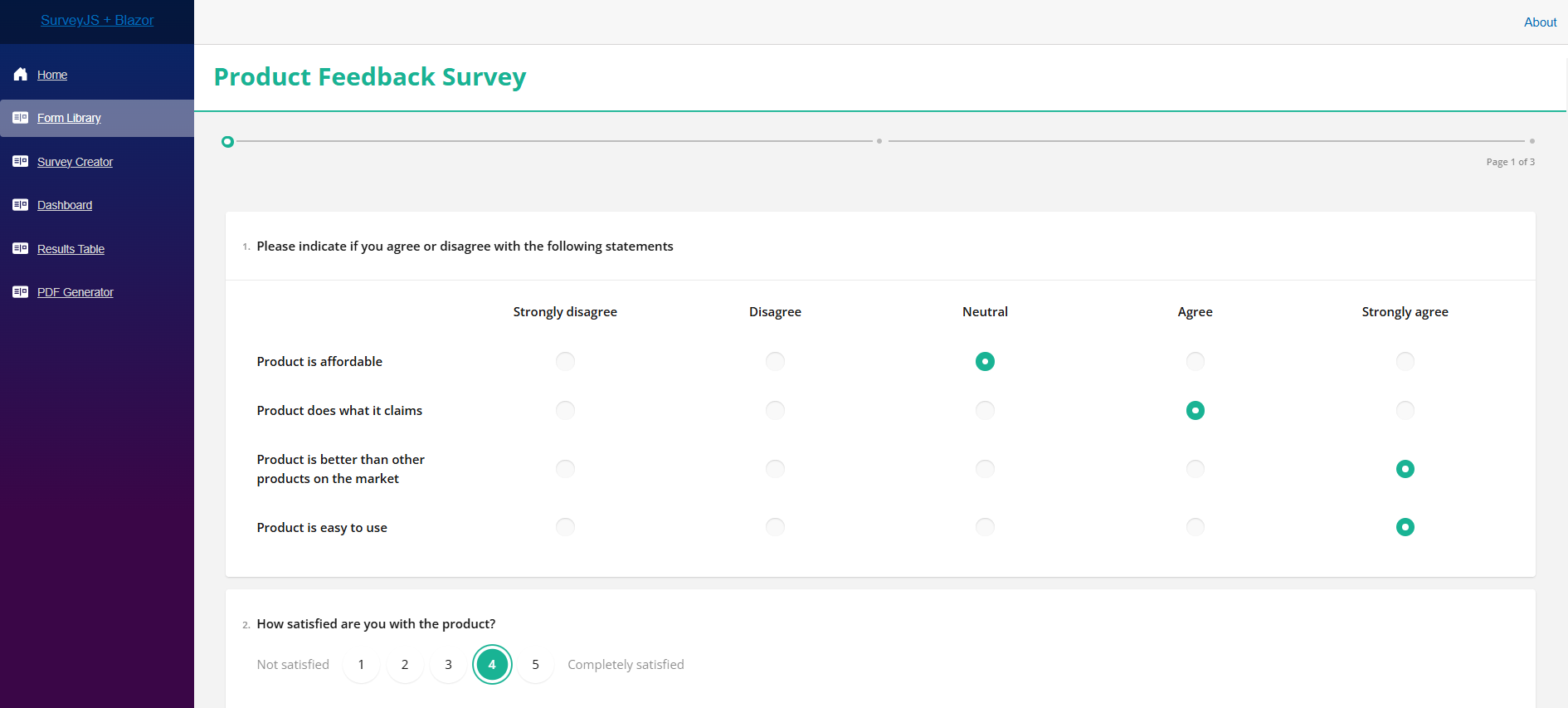
The following code integrates SurveyJS Form Library into a Blazor app. The Survey.tsx
component initializes a form model with a provided JSON schema and renders the form using the Survey
component from the survey-react-ui
package.
// Survey.tsx
import React from "react";
import { Model } from "survey-core";
import { Survey } from "survey-react-ui";
import "survey-core/survey.i18n.js";
import "survey-core/survey-core.css";
export default function SurveyRunner(props: { json?: Object }) {
const model = new Model(props.json);
return (<Survey model={model} />);
}
Get Started with SurveyJS Form Library
Integrate Survey Creator / Form Builder
Survey Creator is a customizable client-side form editor for building surveys and forms in Blazor applications. It automatically generates form definitions (schemas) that describe the form's style, content, layout, and behavior.
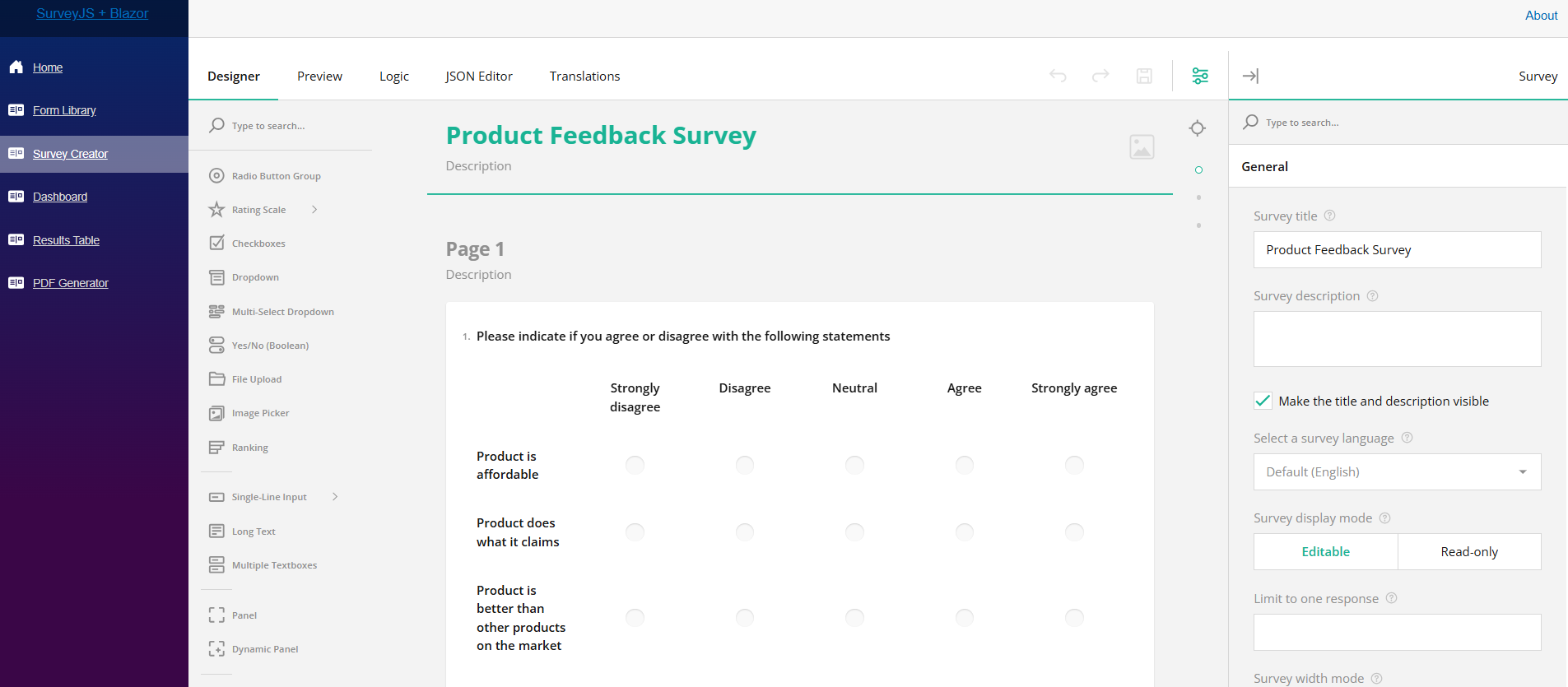
The Survey Creator component is just as easy to integrate using the code below. This code sets up the Survey Creator with default options and allows the user to save the survey JSON schema dynamically.
// Creator.tsx
import React, { useState } from "react";
import { ICreatorOptions } from "survey-creator-core";
import { SurveyCreator, SurveyCreatorComponent } from "survey-creator-react";
import "survey-core/survey.i18n.js";
import "survey-creator-core/survey-creator-core.i18n.js";
import "survey-core/survey-core.css";
import "survey-creator-core/survey-creator-core.css";
const defaultCreatorOptions: ICreatorOptions = {
showTranslationTab: true
};
export default function Creator(props: { json?: Object, options?: ICreatorOptions }) {
let [creator, setCreator] = useState<SurveyCreator>();
if (!creator) {
creator = new SurveyCreator(props.options || defaultCreatorOptions);
creator.saveSurveyFunc = (no: number, callback: (num: number, status: boolean) => void) => {
callback(no, true);
};
setCreator(creator);
}
creator.JSON = props.json;
return (
<SurveyCreatorComponent creator={creator} />
);
}
Get Started with Survey Creator / Form Builder
Integrate Dashboard
SurveyJS Dashboard visualizes survey results using interactive charts and graphs, such as pie charts, bar graphs, and gauges.
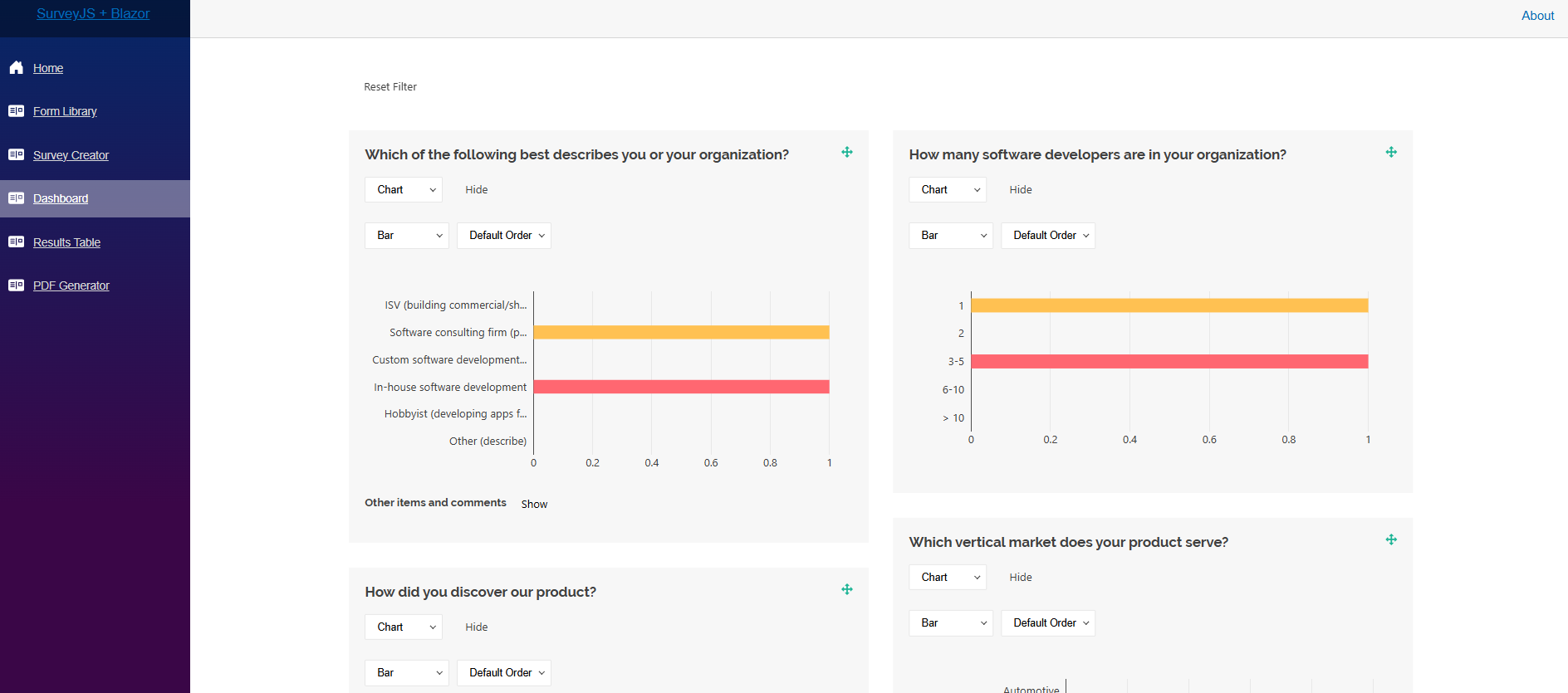
The code below creates a dashboard using the VisualizationPanel
component from the survey-analytics
package. This component requires a list of survey questions that need to be visualized and an array of aggregated answers.
// Dashboard.tsx
import React from "react";
import { useEffect, useState } from "react";
import { Model } from "survey-core";
import { VisualizationPanel } from "survey-analytics";
import "survey-analytics/survey.analytics.css";
import { data, json } from "../../Data/dashboard_data";
export default function Dashboard(param?: any) {
let [vizPanel, setVizPanel] = useState<VisualizationPanel>();
if (!vizPanel) {
const survey = new Model(json);
vizPanel = new VisualizationPanel(survey.getAllQuestions(), data);
setVizPanel(vizPanel);
}
useEffect(() => {
vizPanel?.render("surveyVizPanel");
return () => {
vizPanel?.clear();
}
}, [vizPanel]);
return <div id="surveyVizPanel" style={{"margin": "auto", "width": "100%", "maxWidth": "1400px"}}></div>;
}
Get Started with SurveyJS Dashboard
Integrate Table View for Survey Results
Table View is a UI component that displays users responses in a table. Users can sort, filter, and search within this table to explore the presented dataset.
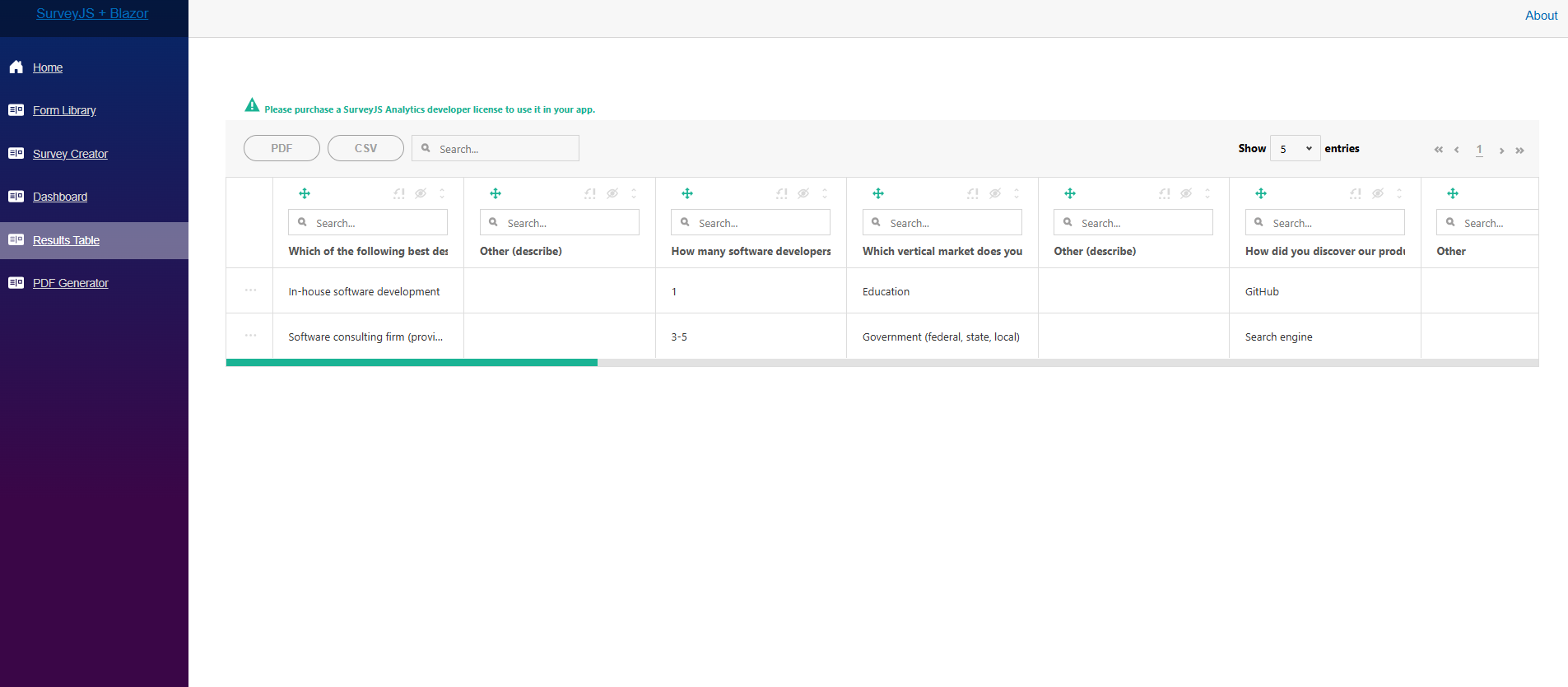
The code below shows how to set up the Table View and integrate it into your Blazor app. This configuration includes the jsPDF and XLSX libraries to let users save the visualized dataset as a PDF or Excel document.
// Tabulator.tsx
import React from "react";
import { useEffect, useState } from "react";
import { data, json } from "../../Data/dashboard_data";
import jsPDF from "jspdf";
import { applyPlugin } from "jspdf-autotable";
applyPlugin(jsPDF);
import * as XLSX from "xlsx";
import { Tabulator } from "survey-analytics/survey.analytics.tabulator.js";
import { SurveyModel } from "survey-core";
import "survey-analytics/survey.analytics.tabulator.css";
import "tabulator-tables/dist/css/tabulator.min.css";
export default function DashboardTabulator(param?: any) {
let [tabulator, setTabulator] = useState<Tabulator>();
if (!tabulator) {
const survey = new SurveyModel(json);
tabulator = new Tabulator(
survey as any,
data,
{ jspdf: jsPDF, xlsx: XLSX }
);
setTabulator(tabulator);
}
useEffect(() => {
tabulator?.render("summaryContainer");
}, [tabulator]);
return <div style={{ height: "80vh", width: "100%" }} id="summaryContainer"></div>;
}
Get Started with Table View for SurveyJS Dashboard
Integrate PDF Generator
SurveyJS PDF Generator is an add-on to the Form Library that enables users to save dynamic surveys as fillable PDF forms for printing, sharing, or offline use. You can customize the page layout and brand the resulting PDF document to generate professional reports.
To generate a PDF form, import the SurveyPDF
object from the survey-pdf
package and pass a survey JSON schema to its constructor. Optionally, you can prefill the PDF form with data. Call the save(fileName)
method to save the PDF form on a user's storage:
import React from "react";
import { Model } from "survey-core";
import { SurveyPDF } from "survey-pdf";
import { json } from "../../Data/survey_json.js";
function savePDF(model: Model) {
const surveyPDF = new SurveyPDF(json);
surveyPDF.data = model.data;
surveyPDF.save();
};
export default function PDFGenerator(param?: any) {
const model = new Model(json);
return (
<div>
<button onClick={() => savePDF(model)}>Save as PDF</button>
</div>
);
}
Get Started with SurveyJS PDF Generator
Conclusion
SurveyJS libraries help you build a complete form management system for Blazor. With the SurveyJS Form Generator, you can quickly design professional, interactive, and visually appealing forms for your web applications. Whether you're creating simple contact forms or complex multi-page surveys, the SurveyJS Blazor form designer provides the necessary tools to simplify your form development.
Use the Form Library to conduct online forms and gather user responses. Visualize data in a table format with the SurveyJS Tabulator or gain valuable insights using the SurveyJS Dashboard. Additionally, easily generate PDF versions of your forms, both empty or prefilled with responses.
To dive deeper, explore the SurveyJS Blazor GitHub repository and begin building your dynamic forms in Blazor today!